Quick tutorial on generating random numbers in Python using the built-in random
module.
1. Import the random
Module:
Python’s random
module provides functions to generate random numbers and perform random operations.
import random
Generating Random Numbers
a. Random Float Between 0 and 1
Generates a random floating-point number between 0 (inclusive) and 1 (exclusive).
print(random.random())
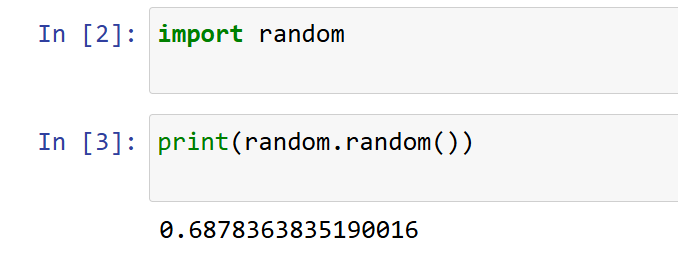
b. Random Integer
Generates a random integer between two specified numbers.
print(random.randint(1, 10)) # Random integer between 1 and 10 (inclusive)

c. Random Float in a Range
Generates a random float between two specified numbers.
print(random.uniform(5, 15)) # Random float between 5 and 15
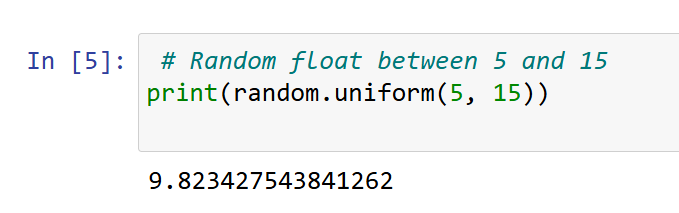
d. Random Numbers in Steps
Choose a random number from a range with a step value.
print(random.randrange(0, 100, 5)) # Random number between 0 and 100, in steps of 5
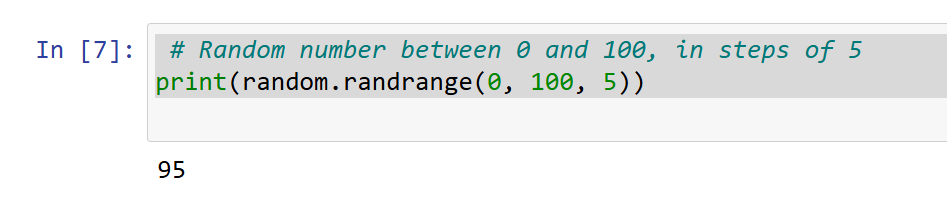
3. Working with Sequences
a. Random Choice
Selects a random element from a sequence (like a list or tuple).
fruits = [‘apple’, ‘banana’, ‘cherry’, ‘date’]
print(random.choice(fruits))
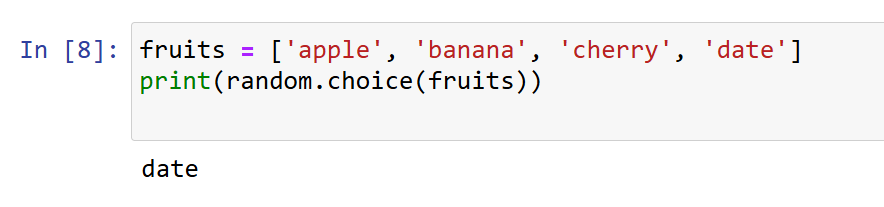
b. Shuffle a List
Shuffles the elements of a list in place.
numbers = [1, 2, 3, 4, 5]
random.shuffle(numbers)
print(numbers)
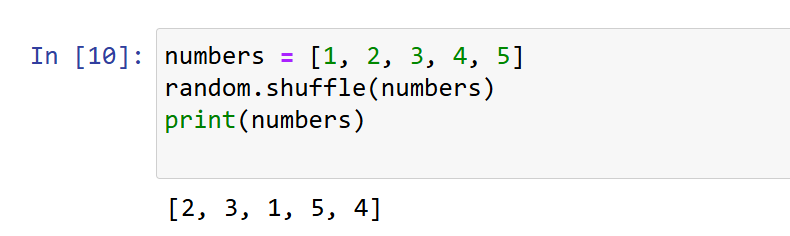
c. Random Sample
Selects a specified number of unique elements from a sequence.
colors = [‘red’, ‘blue’, ‘green’, ‘yellow’, ‘pink’]
print(random.sample(colors, 3)) # Randomly choose 3 unique colors

4. Reproducible Random Numbers
Use a seed to make your random numbers reproducible (useful for debugging).
random.seed(42) # Set the seed
print(random.random()) # This will always generate the same value with the same seed
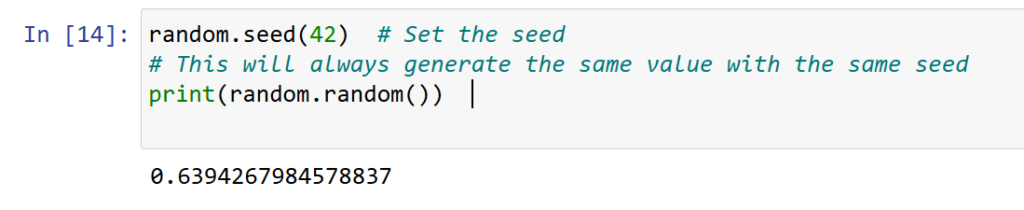
Using numpy
for Random Numbers
If you’re working with large datasets or need advanced random number capabilities, consider using NumPy.
import numpy as np
Generate an array of 5 random integers between 1 and 10
print(np.random.randint(1, 11, 5))
Generate 5 random floats
print(np.random.random(5))
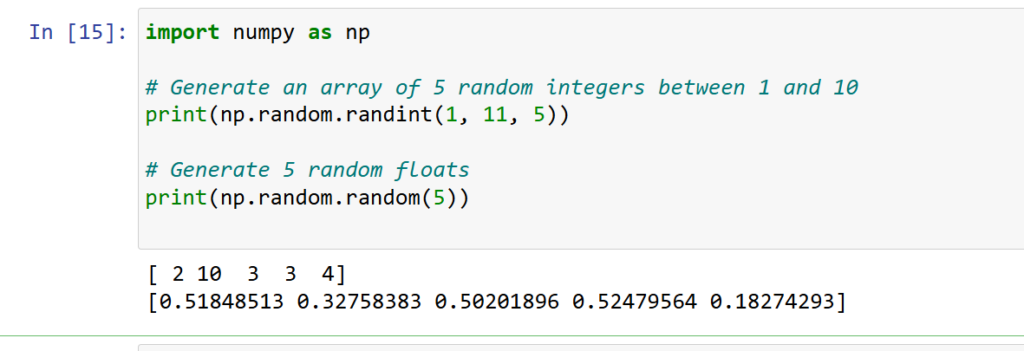
Leave a Reply